Task: Classify Iris Flower#
This Jupyter Notebook is a practical example of how to classify the famous iris flower dataset. The goal is to build a machine learning model that can accurately predict the species of an iris flower based on its measurements.
The iris flower dataset is a well-known dataset in the field of machine learning. It consists of measurements of four features (sepal length, sepal width, petal length, and petal width) of three different species of iris flowers (setosa, versicolor, and virginica). The dataset is commonly used to demonstrate various machine learning algorithms and techniques.
In this notebook, we will walk through the process of loading the iris dataset, visualizing the data, preprocessing the data, building a multilayer perceptron (MLP) model, training the model, evaluating the model’s performance, and visualizing the training progress.
The boilerplate code is already prepared for you!
Let’s get started!
from sklearn.datasets import load_iris
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
import numpy as np
iris = load_iris()
plt.scatter(iris.data[:, 0], iris.data[:, 1], c=iris.target)
plt.xlabel('Sepal Length')
plt.ylabel('Sepal Width')
plt.title('Iris Dataset')
plt.show()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2, random_state=42)
print(iris.data.shape)
print('Train:', X_train.shape, y_train.shape)
print('Test:', y_train.shape, y_test.shape)
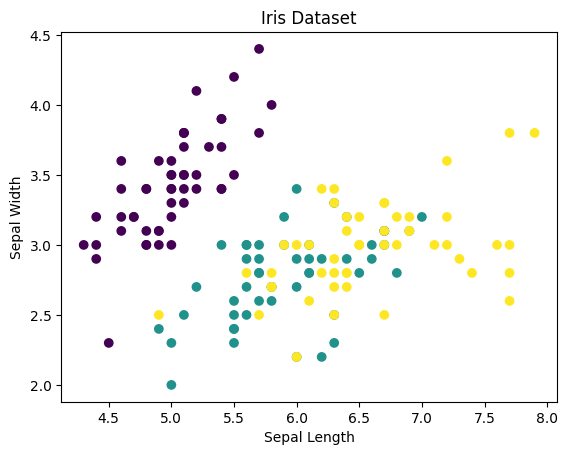
(150, 4)
Train: (120, 4) (120,)
Test: (120,) (30,)
Questions & Tasks#
How many classes do we have?
How many features do we have?
Use the Multilayer Perceptron. We need to modify the Input and Output Layer as well as the Learning Rate. Explain the changes.
What is the accuracy of the MLP model?
How many epochs did the training loop run for?
What is the purpose of the Sigmoid activation function?
How does the Softmax activation function work?
Can you visualize the training progress?
Can you create a confusion matrix for the MLP model? What is that?
# I have already prepared the softmax.
class Softmax:
def forward(self, input):
self.result = np.exp(input)/np.sum(np.exp(input))
return self.result
def backward(self, targets, _):
return self.result - targets
# Copy MLP here and adapt it, you find it in the mlp.ipynb file
# network = Network(...)
# network.forward(X_train[0])
epochs = 1000
losses = []
# Finish the training loop
for epoch in range(epochs):
loss = 0
for x, y in zip (X_train, y_train):
pass
losses.append(loss)
correct_predictions = 0
total_predictions = len(X_test)
predictions = []
for x, y in zip(X_test, y_test):
# prediction = network.forward(x)
# correct_predictions += np.argmax(prediction) == y
pass
accuracy = correct_predictions / total_predictions
# print("Accuracy: ", accuracy)
# plt.plot(losses)
# Draw a confusion matrix
# Hint use sklearn.metrics.confusion_matrix and sklearn.metrics.ConfusionMatrixDisplay